It is no exaggeration to say that in the Humble Framework, there's a lot going on in the controllers.
The controllers are XML files that handle routing, data scrubbing, data validation, object allocations, and even event triggering. These controllers get compiled to PHP
programs and placed in the
Cache folder within the same directory that XML files reside.
Important!
When creating a controller,
Always use the wizards in the admin section. Do not create one from scratch.
Pro-Tip: Customize your creations
When you installed Humble, in your primary application module a lib directory was copied from the base Humble module.
In this directory you will find the code skeletons that will be used when creating components and modules. You can edit these skeletons to add comments, license information, ASCII art,
logos, etc... In fact, when you create a component you have to identify the namespace that the component belongs to, and if the module managed by that namespace has a lib
folder, those skeletons will be used when creating the component, so it is possible to have different licensing, logo, or anything else per module.
In the administration section, there is a wizard at the bottom that allows you to create a controller, along with the first action in that controller. Besides naming the controller and providing
the descriptions, you will need to identify the default templating engine this controller will use, and you will need to do this even if you plan on only outputting JSON.
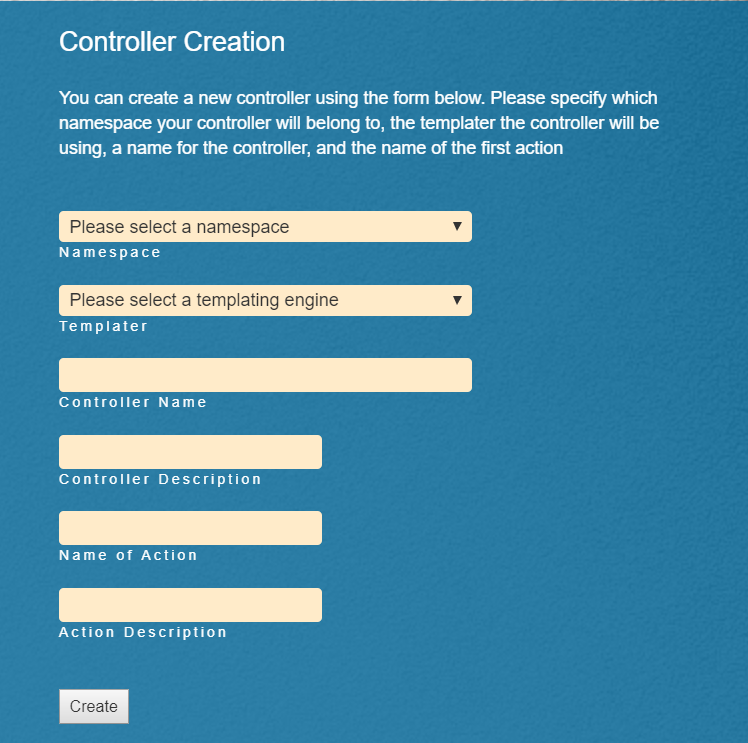
Wizard
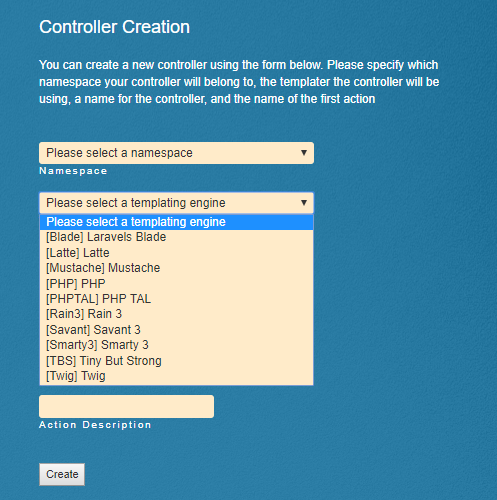
Available Templating Engines
After you have created your controller, there will be a new folder with the same name as the controller in the Views directory of the module identified by the namespace used during controller creation.
In the new folder you will find a folder with the name of the templating engine selected, and an empty file with the name of the action you created, using the appropriate file extension.
For reference, we are going to use this simple controller below:
A controller contains one to many actions. Each action has at a minimum a name which constitutes the third segment in our routing URI scheme (see
Routing Basics).
Each action may, or may not, have a dedicated view, which will be found in the Views folder, in a folder named the same as this controller.
By using the
<view name="other_view"> directive, you can override the default view handling.
Here is an example of handling a request: A URI of
/acme/customer/list would look for the controller in the 'Controllers' directory of the module identified by the 'acme' namespace.
Once found and compiled if necessary,the framework will then look for an action called 'list' in the compiled controller, execute the code found there, and then route to a view with the name of 'list'.
So the request URI of
/acme/customer/list would use the following two files:
- Controller: /app/Code/package/Acme/Controllers/customer.xml
- View File: /app/Code/package/Acme/Views/customer/Twig/list.twig
The above example assumes you are using the Twig templating engine.
Each action begins with the XML tag "action" followed by the required "name" attributed, and 0 to many additional attributes. These additional attributes control high-level features of the actions behavior. A list of
additional attributes follows (in no particular order):
- output - The Content-Type of the response. Default is HTML, but can identify JSON, PDF, CSV, or any valid mime-type.
- blocking - Values On/Off - whether this particular action will "block" on Session variables. If you don't need to write to the session, turning blocking off can increase
performance significantly under many cases.
- namespace/class - This is an alternate way of processing, if you specify the namespace of a module, and the classname of a Model within that module, prior to being handed to the view,
a method named "execute()" in that Model will be called and that Model will have access to all resources that were defined in that action. This kind of follows an old Java/Struts pattern.
- event/comment - This registers an event in the Paradigm Engine with the descriptive comment indicated. From within the Paradigm Engine, you can then design workflows that "listen"
for that event, and each time this action is called an Event of that event type will be emitted, a long with all the data that was identifed on the request with a parameter tab or passalong attribute.
Request variables that were not in some way parameterized will not be passed with the Event.
The following are most (but not necessarily all) of the elements that can comprise an Action
- description - A description of this actions purpose. This description is used in a Searchable Directory so please include it and make meaningful. The directory is available from the admin page.
- model/helper/entity - These are the primary resources of your application, and are covered later in this document
- switch/if - Two mechanisms for selective processing, covered later in this document
- view - An override mechanism so you can share views between actions
- chain - Identifies a series of 1 or more actions to perform after this action. This is how we achieve Daisy-Chaining of actions.
- redirect - When you need to go to an action in another controller, or access some resource not a component of the current namespace, then use a redirect. Otherwise use a "chain".
- output - Outputs some static text, used when a cheap and simple mechanism is all you need. Don't make too much use of this...
- abort - Most often used with "switch/if", will force an abrupt end to processing and prevent any additional bubbling of events.
A resource is a reference to a PHP class. There are only three kinds of resources that are readily available in your Controller, and these are listed below
- Model - A class containing custom logic, usually centered on processes. These can contain workflow components
- Helper - A class containing utility methods. These classes are allocated as Singletons and should not attempt to maintain state, also they may not contain workflow components
- Entity - A class representing a DB Table/Collection. The class must follow the naming conventions related to Entities, and these are not allowed to contain workflow components